Getting started
Step 1: Setup the development environment​
What you need before starting​
- Your AWS account is SSO-enabled to access https://deltatre.awsapps.com/start. After being SSO-enabled (please refer to your Project Manager), restart your development machine.
Setup​
- Access https://deltatre.awsapps.com/start and select
Access keys
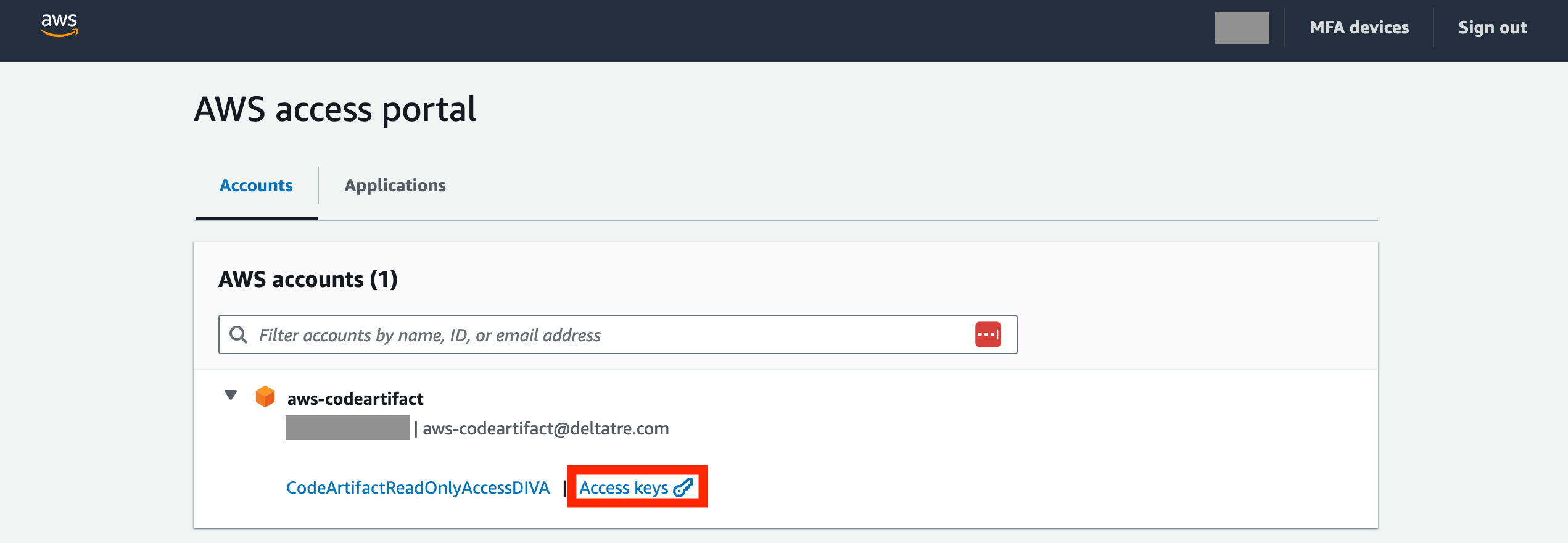
- In a terminal, execute the three imports in
Option 1: Set AWS environment variables
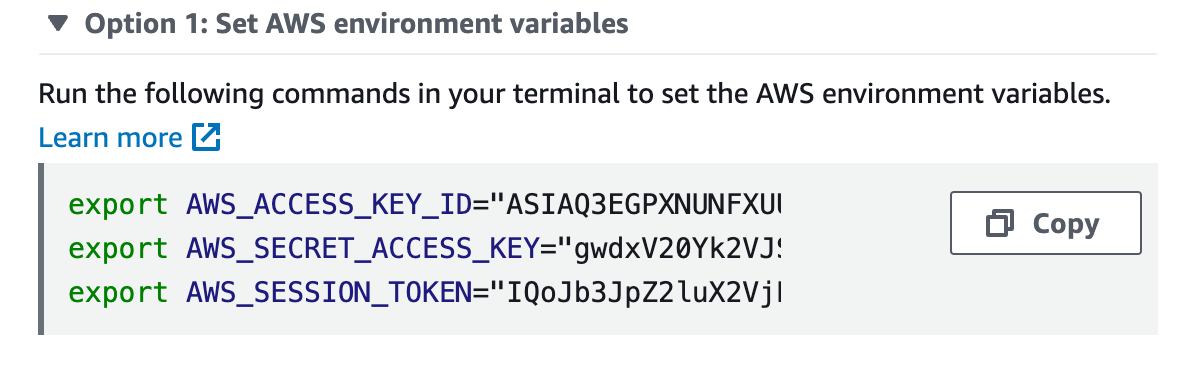
- Go back to https://deltatre.awsapps.com/start and select
CodeArtifactReadOnlyAccessDIVA
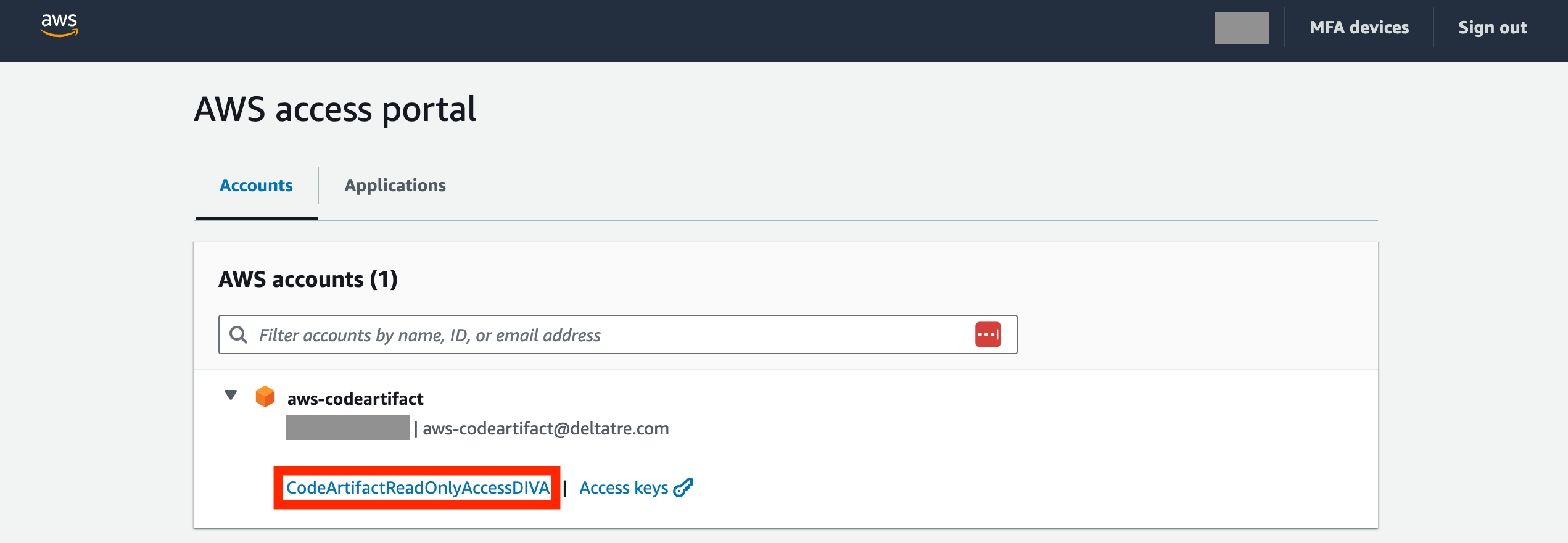
- Search and select
CodeArtifact
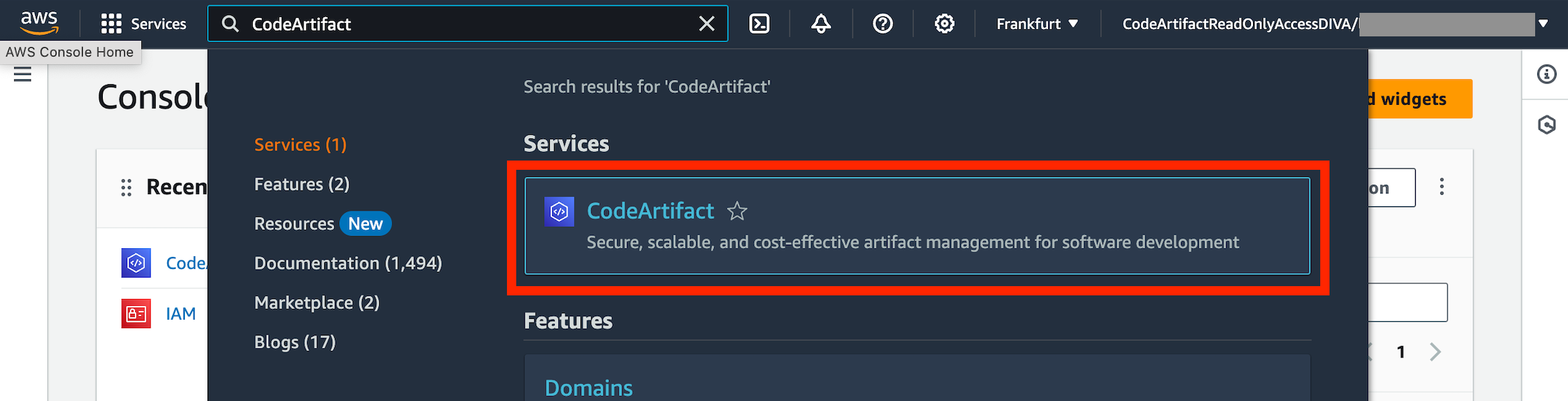
- Select the
Diva
repository, filter byFormat:generic
, and selectView connection instructions
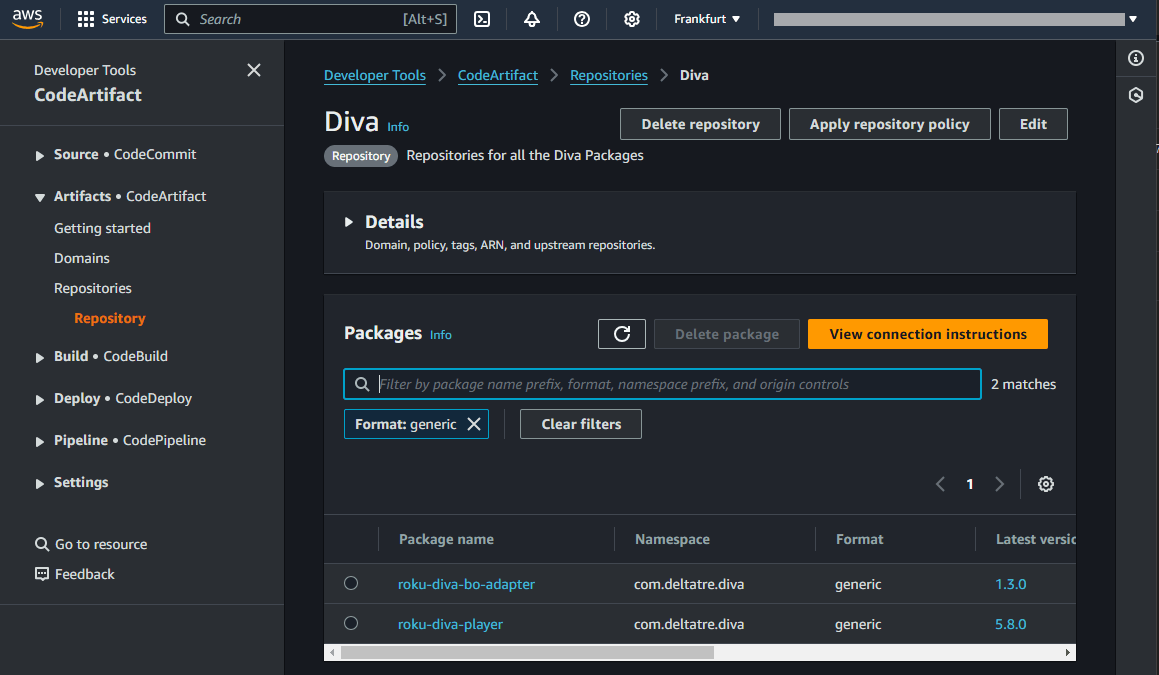
- Select
Operating system
,npm
as the package manager,Recommended method: configure using AWS CLI
, and copy the login command in Step 3
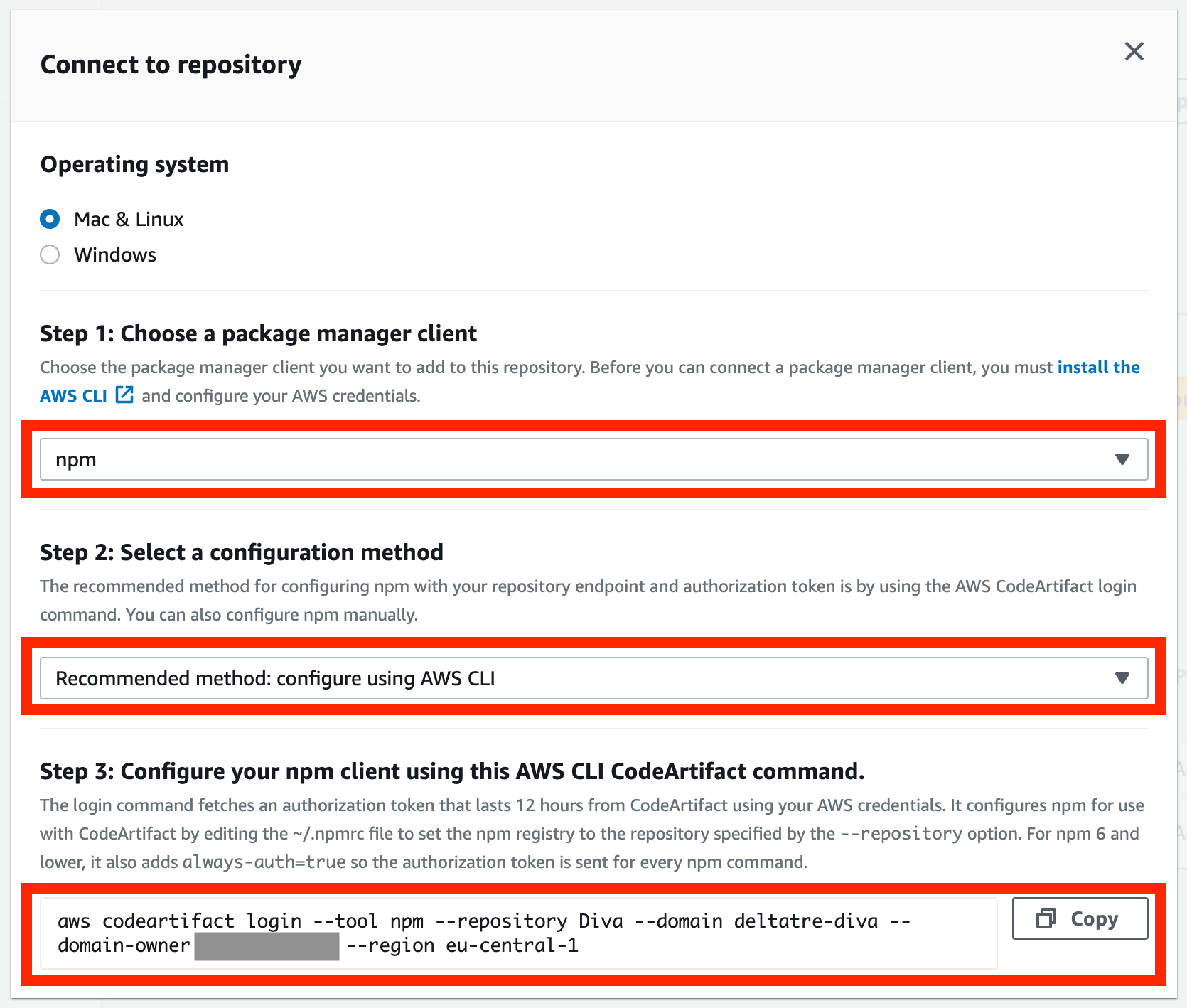
Note: The login session expires within 12 hours. Repeat the steps until the login command every time you need to update the DIVA package.
- Download the
Diva Player lib
andDIVA Back Office Adapter lib
from the AWS CodeArtifact.
Diva Player lib
you can find in AWS CodeArtifact by Package name
- roku-diva-player
DIVA Back Office Adapter lib
you can find in AWS CodeArtifact by Package name
- roku-diva-bo-adapter
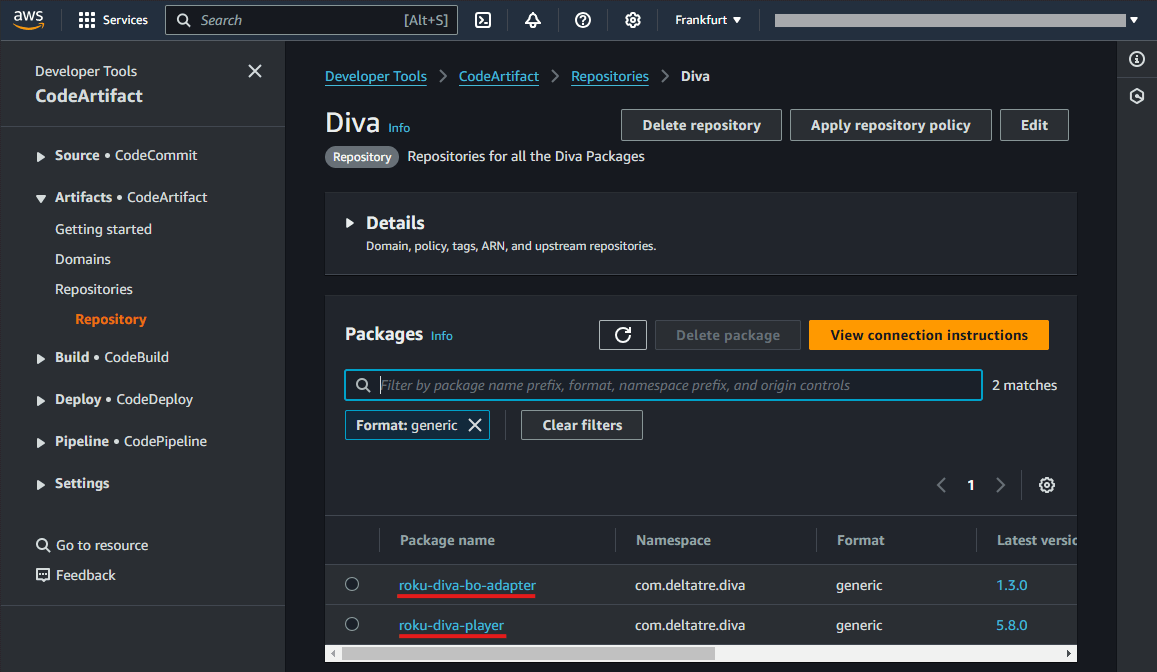
The approach for downloading Diva Player lib
and DIVA Back Office Adapter lib
are identical.
The next steps 8-12 describe how to download the Diva Player lib
and these steps are similar to downloading the DIVA Back Office Adapter lib
- Find the package in AWS CodeArtifact. Navigate to the
Diva
repository. Add package filter by name:roku-diva-player
/roku-diva-bo-adapter
Node: roku-diva-player
for Diva Player lib
. roku-diva-bo-adapter
for DIVA Back Office Adapter lib

- Select the
roku-diva-player
/roku-diva-bo-adapter
package in the search result

- On the Package page select the required Package version
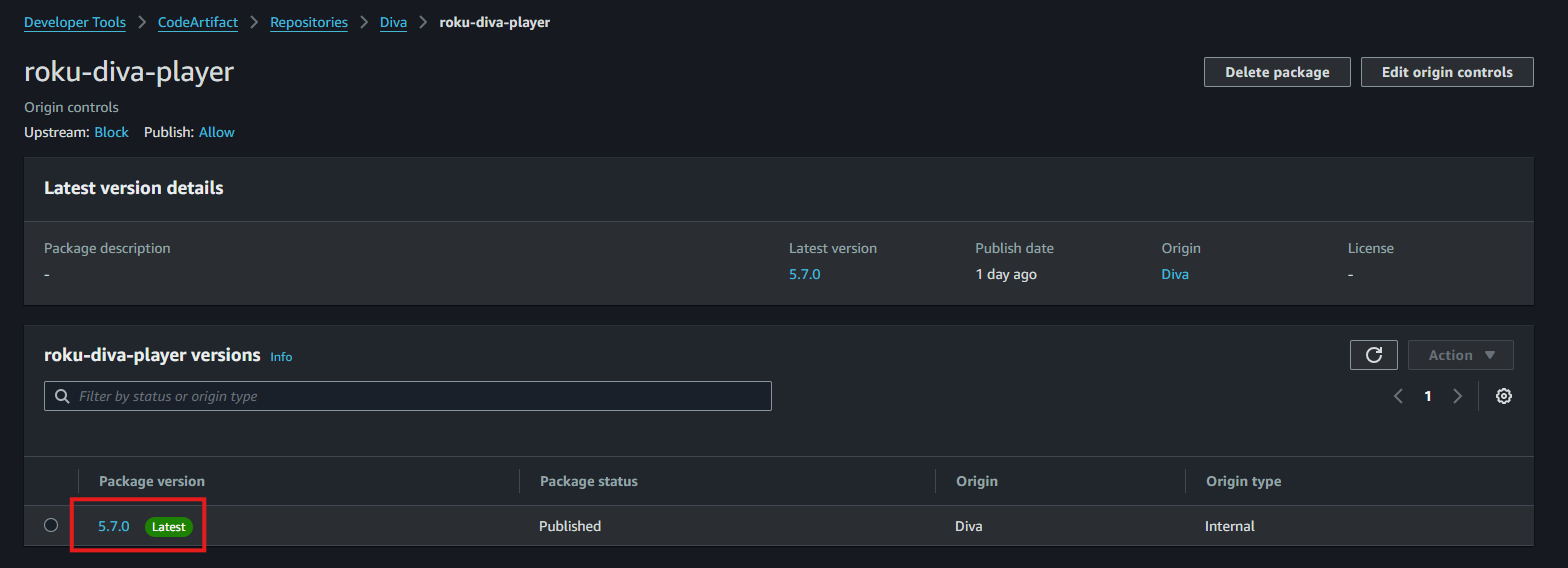
- At the Asset page find the
package name
(1),version
(2) andpackage lib name
(3)
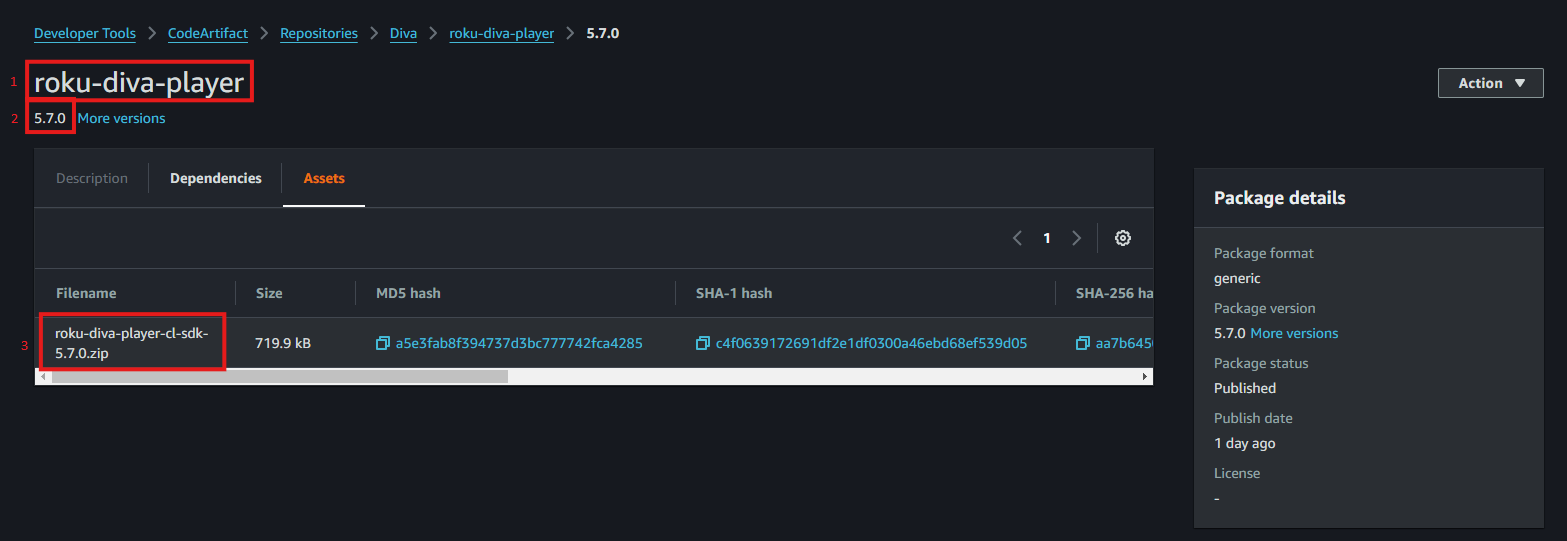
- Download package (
roku-diva-player
/roku-diva-bo-adapter
). Perform commandaws codeartifact get-package-version-asset
in the cmd line:
Note: This command for cmd line on Windows, for other platform use AWS instruction here
Replace values in command before sending:
aws codeartifact get-package-version-asset --domain deltatre-diva --repository Diva ^
--format generic --namespace com.deltatre.diva --package PACKAGE_NAME ^
--package-version PACKAGE_VERSION --asset PACKAGE_LIB_NAME PACKAGE_LIB_NAME
PACKAGE_NAME
- it is the package name
(1) from the Step 11. Can be: roku-diva-player
/ roku-diva-bo-adapter
PACKAGE_VERSION
it is the version
(2) from the Step 11. For example: 5.7.0
PACKAGE_LIB_NAME
it is the package lib name
(3) from the Step 11. For example: roku-diva-player-cl-sdk-5.7.0.zip
Example of command for downloading the roku-diva-player
(Diva Player lib
):
aws codeartifact get-package-version-asset --domain deltatre-diva --repository Diva ^
--format generic --namespace com.deltatre.diva --package roku-diva-player ^
--package-version 5.7.0 --asset roku-diva-player-cl-sdk-5.7.0.zip roku-diva-player-cl-sdk-5.7.0.zip
Example of command for downloading the roku-diva-bo-adapter
(DIVA Back Office Adapter lib
):
aws codeartifact get-package-version-asset --domain deltatre-diva --repository Diva ^
--format generic --namespace com.deltatre.diva --package roku-diva-bo-adapter ^
--package-version 1.2.0 --asset roku-diva-bo-adapter-cl-1.2.0.zip roku-diva-bo-adapter-cl-1.2.0.zip
-
Store the
Diva Player Lib
andDIVA DIVA Back Office Adapter Lib
in your Roku project folder in thepkg:/resources/...
folder -
After downloading the
Diva Player Lib
andDIVA DIVA Back Office Adapter Lib
you are ready to create the first Roku app with DIVA Player.
Step 2: Write your very first Roku app with DIVA Player​
What you need before starting​
- Ensure you download the
Diva Player Lib
andDIVA DIVA Back Office Adapter Lib(roku-diva-bo-adapter)
from the Setup section of this instruction. - Ask your video engineers team the
<video id>
and<settings URL>
.
Instantiation​
Replace <Diva Player lib path>
and <DIVA Back Office Adapter lib path>
with paths where you stored the libs (Setup > Step 13),
Replace <video id>
and <settings URL>
with values you got from your video engineers team in the following code:
sub init()
initScreen()
end sub
sub initScreen()
loadLibs()
end sub
sub loadLibs()
'Init of loading the Diva Player lib
loadPlayer()
'Init of loading the DIVA Back Office Adapter lib
loadBOAdapter()
end sub
sub loadPlayer()
' Creates the ComponentLibrary (the DivaPlayerSDK in this case)
m.divaPlayerSDK = CreateObject("roSGNode", "ComponentLibrary")
m.divaPlayerSDK.id = "DivaPlayerSDK"
m.divaPlayerSDK.uri = "<Diva Player lib path>"
' Adding the ComponentLibrary node to the scene will start the download of the library
m.top.appendChild(m.divaPlayerSDK)
m.divaPlayerSDK.observeField("loadStatus", "onDivaPlayerLoadStatusChanged")
end sub
sub loadBOAdapter()
' Creates the ComponentLibrary (the DIVA Back Office Adapter in this case)
m.divaBOAdapter = CreateObject("roSGNode", "ComponentLibrary")
m.divaBOAdapter.id = "DivaBOAdapter"
m.divaBOAdapter.uri = "<DIVA Back Office Adapter lib path>"
' Adding the ComponentLibrary node to the scene will start the download of the library
m.top.appendChild(m.divaBOAdapter)
m.divaBOAdapter.observeField("loadStatus", "onDivaPlayerLoadStatusChanged")
end sub
sub onDivaPlayerLoadStatusChanged()
if m.divaPlayerSDK.loadStatus = "ready"
if m.divaBOAdapter <> invalid and m.divaBOAdapter.loadStatus = "ready"
launchBOAdapter()
end if
end if
end sub
sub launchBOAdapter()
m.boAdapterNode = CreateObject("roSGNode", "DivaBOAdapter:DivaBOAdapter")
m.boAdapterNode.initData = {
"settingId": "<settings URL>"
"videoId": "<video id>"
"dictionaryId": "en_GB"
}
' Observe DIVA Back Office Adapter DiveLaunchParams
m.boAdapterNode.observeField("divaLaunchParams", "onBOAdapterDivaLaunchParamsHandler")
' Observe DIVA Back Office Adapter Dictionary
m.boAdapterNode.observeField("dictionary", "onBOAdapterDictionaryHandler")
' Observe DIVA Back Office Adapter VideoMetaDataNode
m.boAdapterNode.observeField("videoDataNode", "onBOAdapterVideoDataNodeHandler")
' Observe DIVA Back Office Adapter Entitlement Data
m.boAdapterNode.observeField("entitlementData", "onEntitlementDataHandler")
' Observe DIVA Back Office Adapter Settings
m.boAdapterNode.observeField("settingsNode", "onSettingsHandler")
' Observe DIVA Back Office Adapter when all necessary data for launching the Diva Player was loaded
' After getting this event you can launch the Diva Player
m.boAdapterNode.observeField("boAdapterReady", "onBOAdapterReady")
'Diva Player Utils Node
m.dpUtilsNode = getDivaPlayerUtilsNode()
' Observe Diva Player exit call
m.dpUtilsNode.observeField("divaPlayerExit", "onDivaPlayerExitHandler")
' Observe Diva Player actions
' Observe Diva Metadata update call
m.dpUtilsNode.observeField("metaDataUpdate", "onMetadataUpdateHandler")
' Get a request from the Diva Player on making the Entitlement request to the BE
m.dpUtilsNode.observeField("entitlementRequest", "onEntitlementHandler")
' Getting Diva Player Node
m.divaPlayer = m.dpUtilsNode.callFunc("getPlayer")
' Adding Diva Player for the visualisation
screensStack = m.getScreensStack()
screensStack.appendChild(m.divaPlayer)
m.divaPlayer.setFocus(true)
end sub
function getDivaPlayerUtilsNode() as dynamic
if NOT isValid(m.dpUtilsNode)
m.dpUtilsNode = CreateObject("roSGNode", "DivaPlayerSDK:DPUtilsNode")
end if
return m.dpUtilsNode
end function
sub onBOAdapterDivaLaunchParamsHandler(evt as dynamic)
data = evt.getData()
' Setup Diva Player launch parameters
m.dpUtilsNode.callFunc("setLaunchParams", data)
end sub
sub onBOAdapterDictionaryHandler(evt as dynamic)
data = evt.getData()
' Setup Diva Player dictionary
m.dpUtilsNode.callFunc("setDictionary", data)
end sub
sub onBOAdapterVideoDataNodeHandler(evt as dynamic)
data = evt.getData()
' Setup Diva Player Metadata
m.dpUtilsNode.callFunc("setMetaData", data)
end sub
sub onSettingsHandler(evt as dynamic)
data = evt.getData()
' Setup Diva Player Settings
m.dpUtilsNode.callFunc("setSettings", data)
end sub
sub onEntitlementDataHandler(evt as dynamic)
' Set to the Diva Player response from Entitlement Service
m.dpUtilsNode.callFunc("setEntitlementData", evt.getData())
end sub
sub onBOAdapterReady(evt as dynamic)
m.dpUtilsNode.callFunc("runPlayer")
end sub
sub destroyBOAdapter()
if m.boAdapterNode <> invalid
m.boAdapterNode.unobserveField("error")
m.boAdapterNode.destroy = true
m.boAdapterNode = invalid
end if
end sub
sub onMetadataUpdateHandler(evt as dynamic)
data = evt.getData()
m.boAdapterNode.requestMetaDataUpdate = data
end sub
sub onEntitlementHandler(evt as dynamic)
data = evt.getData()
m.boAdapterNode.entitlementRequest = data
end sub
sub onDivaPlayerExitHandler()
m.dpUtilsNode = invalid
'Destroy DIVA Back Office Adapter Node
destroyBOAdapter()
end sub